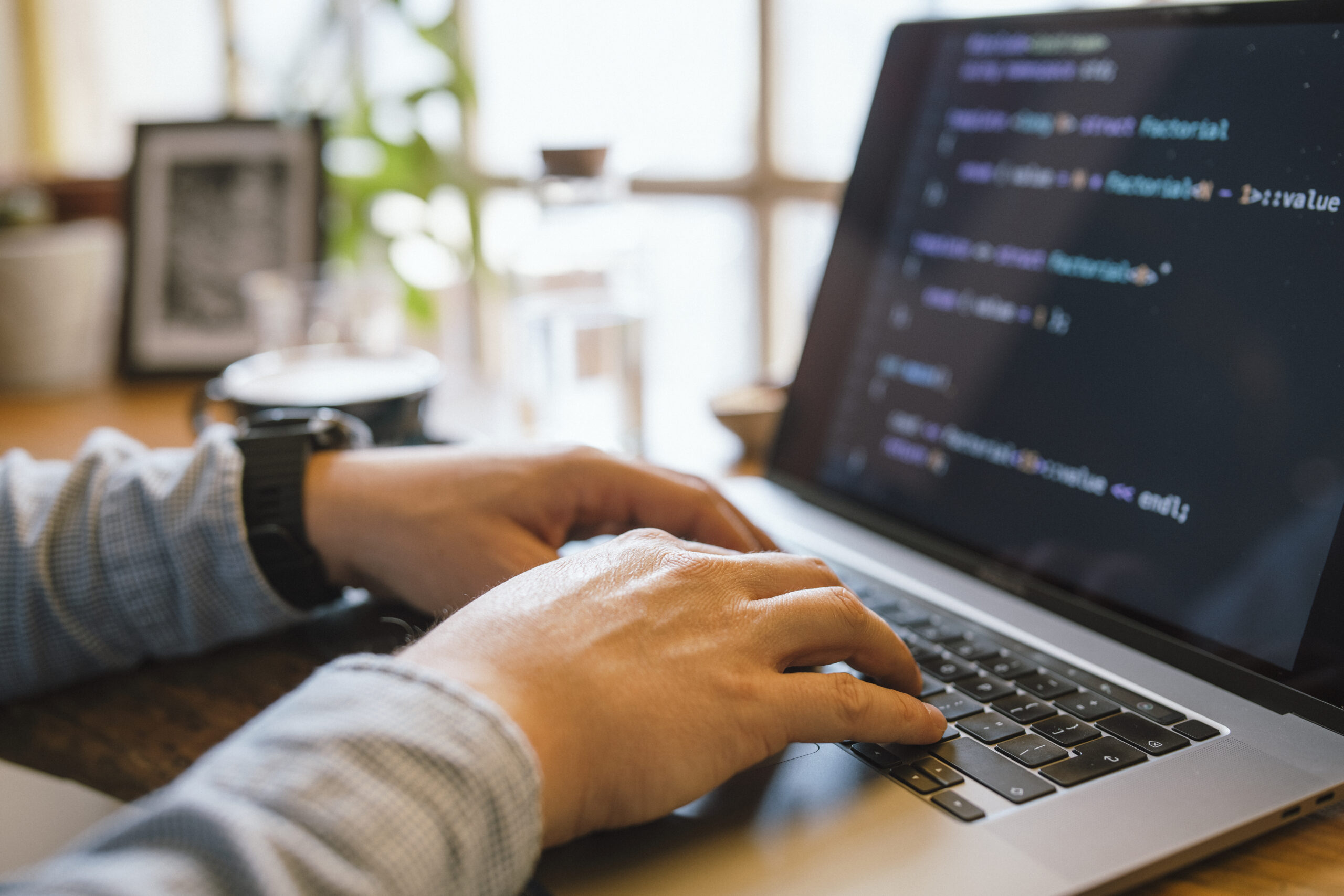
Debugging is Among the most essential — nevertheless usually forgotten — competencies in a very developer’s toolkit. It isn't really just about fixing damaged code; it’s about comprehending how and why issues go Completely wrong, and learning to think methodically to solve problems efficiently. Regardless of whether you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and dramatically improve your efficiency. Here i will discuss numerous techniques to assist developers level up their debugging game by me, Gustavo Woltmann.
Learn Your Instruments
Among the list of quickest techniques developers can elevate their debugging abilities is by mastering the tools they use every day. Though producing code is a single Section of advancement, realizing how to connect with it properly in the course of execution is equally essential. Fashionable progress environments appear Outfitted with potent debugging abilities — but lots of builders only scratch the surface of what these instruments can do.
Choose, one example is, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, phase through code line by line, and in many cases modify code around the fly. When made use of appropriately, they Permit you to notice precisely how your code behaves all through execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, including Chrome DevTools, are indispensable for front-conclude builders. They let you inspect the DOM, observe network requests, watch true-time performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can change disheartening UI difficulties into workable tasks.
For backend or technique-amount developers, resources like GDB (GNU Debugger), Valgrind, or LLDB present deep Handle around operating procedures and memory administration. Studying these equipment could possibly have a steeper Understanding curve but pays off when debugging effectiveness issues, memory leaks, or segmentation faults.
Past your IDE or debugger, turn into at ease with version Command methods like Git to comprehend code heritage, obtain the precise moment bugs were launched, and isolate problematic variations.
Ultimately, mastering your tools indicates going past default settings and shortcuts — it’s about producing an personal expertise in your improvement surroundings to ensure when difficulties arise, you’re not lost in the dark. The better you know your tools, the more time you can devote fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
The most essential — and sometimes disregarded — measures in successful debugging is reproducing the trouble. Just before leaping in to the code or creating guesses, developers require to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of probability, normally resulting in wasted time and fragile code variations.
Step one in reproducing an issue is accumulating as much context as you can. Ask thoughts like: What actions triggered The problem? Which environment was it in — enhancement, staging, or output? Are there any logs, screenshots, or error messages? The greater element you've got, the easier it will become to isolate the exact conditions underneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your local setting. This could indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking method states. If The problem seems intermittently, contemplate producing automated exams that replicate the sting circumstances or point out transitions involved. These checks not only support expose the situation but also avoid regressions in the future.
Often, The difficulty might be setting-unique — it might take place only on certain working programs, browsers, or less than particular configurations. Making use of instruments like Digital equipment, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a frame of mind. It involves persistence, observation, plus a methodical technique. But when you finally can consistently recreate the bug, you're presently halfway to repairing it. With a reproducible scenario, You can utilize your debugging equipment far more proficiently, take a look at opportunity fixes safely, and communicate much more clearly together with your group or customers. It turns an abstract complaint into a concrete challenge — and that’s exactly where developers thrive.
Study and Comprehend the Error Messages
Error messages are often the most valuable clues a developer has when something goes wrong. Rather then looking at them as discouraging interruptions, builders really should study to deal with error messages as immediate communications with the technique. They usually inform you just what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start off by looking through the message diligently As well as in total. Many builders, especially when less than time pressure, look at the primary line and right away start building assumptions. But deeper during the error stack or logs might lie the true root lead to. Don’t just copy and paste mistake messages into search engines like yahoo — browse and recognize them first.
Split the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it position to a specific file and line number? What module or operate triggered it? These inquiries can guide your investigation and level you towards the responsible code.
It’s also handy to grasp the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Understanding to acknowledge these can substantially increase your debugging procedure.
Some glitches are imprecise or generic, and in Individuals conditions, it’s essential to examine the context by which the error transpired. Look at associated log entries, input values, and recent improvements in the codebase.
Don’t forget compiler or linter warnings possibly. These often precede bigger troubles and supply hints about opportunity bugs.
Ultimately, error messages usually are not your enemies—they’re your guides. Learning to interpret them effectively turns chaos into clarity, encouraging you pinpoint issues quicker, minimize debugging time, and become a far more successful and self-confident developer.
Use Logging Wisely
Logging is Just about the most strong equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an application behaves, helping you comprehend what’s happening under the hood without having to pause execution or move in the code line by line.
A very good logging system starts with understanding what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for in depth diagnostic information and facts all through progress, Details for standard activities (like effective start-ups), Alert for potential challenges that don’t split the application, Mistake for genuine troubles, and FATAL in the event the process can’t go on.
Prevent flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Center on crucial functions, state improvements, input/output values, and important determination points in the code.
Format your log messages Evidently and persistently. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) can make it even simpler to parse and filter logs programmatically.
For the duration of debugging, logs let you observe how variables evolve, what conditions are fulfilled, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments wherever stepping via code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with monitoring dashboards.
In the long run, wise logging is about stability and clarity. Which has a properly-assumed-out logging strategy, you could reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized process—it is a method of investigation. To properly detect and fix bugs, developers need to technique the procedure similar to a detective resolving a mystery. This state of mind can help stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Start out by accumulating evidence. Look at the signs of the trouble: error messages, incorrect output, or functionality difficulties. The same as a detective surveys against the law scene, accumulate just as much related details as you'll be able to without having jumping to conclusions. Use logs, check instances, and user reports to piece alongside one another a transparent picture of what’s going on.
Future, sort hypotheses. Check with on your own: What may be triggering this conduct? Have any adjustments lately been created towards the codebase? Has this problem happened in advance of beneath equivalent situations? The goal should be to slim down prospects and discover prospective culprits.
Then, test your theories systematically. Seek to recreate the situation in the controlled ecosystem. In case you suspect a particular functionality or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code concerns and Enable the outcome lead you nearer to the truth.
Fork out shut consideration to small facts. Bugs usually disguise while in the least envisioned areas—similar to a missing semicolon, an off-by-one particular error, or possibly a race situation. Be complete and affected person, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may well cover the actual difficulty, just for it to resurface later.
And finally, continue to keep notes on Whatever you tried using and discovered. Just as detectives log their investigations, documenting your debugging course of action can preserve time for future issues and support others recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach difficulties methodically, and grow to be simpler at uncovering concealed issues in complicated programs.
Generate Tests
Creating exams is one of the best tips on how to increase your debugging techniques and In general improvement efficiency. Exams not merely enable capture bugs early but will also function a security Web that gives you self-assurance when generating improvements on your codebase. A perfectly-analyzed application is simpler to debug since it permits you to pinpoint just the place and when a dilemma takes place.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can swiftly reveal regardless of whether a certain bit of logic is Functioning as anticipated. Whenever a test fails, you immediately know where to glimpse, noticeably cutting down enough time invested debugging. Unit tests are especially practical for catching regression bugs—difficulties that reappear soon after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclusion checks into your workflow. These aid make certain that various portions of your application work with each other effortlessly. They’re notably helpful for catching bugs that occur in advanced techniques with several components or expert services interacting. If one thing breaks, your checks can inform you which part of the pipeline unsuccessful and below what ailments.
Composing checks also forces you to Imagine critically regarding your code. To test a aspect effectively, you would like to grasp its inputs, expected outputs, and edge situations. This level of comprehension naturally sales opportunities to raised code construction and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug is often a powerful initial step. When the test fails constantly, you could give attention to repairing the bug and check out your check go when the issue is settled. This tactic ensures that the identical bug doesn’t return Sooner or later.
In brief, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable process—aiding you capture additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult challenge, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Answer right after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, cut down frustration, and often see the issue from the new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief wander, a espresso split, and even switching to a special job for ten–quarter-hour can refresh your target. Quite a few developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, In particular for the duration of for a longer time debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you in advance of.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–10 moment crack. Use that time to maneuver about, extend, or do anything unrelated to code. It may come to feel counterintuitive, especially beneath limited deadlines, nonetheless it in fact leads to more rapidly and more practical debugging Eventually.
To put it briefly, using breaks will not be a sign of weak point—it’s a wise approach. It presents your brain Room to breathe, increases your perspective, and aids you steer clear of the tunnel eyesight that often blocks your progress. Debugging is often a mental puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Every bug you experience is much more than simply A short lived setback—it's an opportunity to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing useful when you make an effort to reflect and examine what went Mistaken.
Start out by inquiring you a few important concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it are already caught previously with superior techniques like device screening, code testimonials, or logging? The responses often expose blind places in the workflow or being familiar with and assist you Develop stronger coding routines moving forward.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s through a Slack information, a short create-up, or A fast expertise-sharing session, assisting others steer clear of the identical issue boosts staff efficiency and cultivates a much better Finding out culture.
Extra importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. Rather than dreading bugs, you’ll begin appreciating them as critical areas of your development journey. All things considered, a few of the most effective developers are certainly not the ones who produce ideal code, but people that constantly study from their errors.
In the long run, Every bug you correct provides a fresh click here layer towards your skill established. So subsequent time you squash a bug, have a instant to reflect—you’ll arrive absent a smarter, more able developer as a result of it.
Summary
Improving your debugging expertise usually takes time, practice, and persistence — although the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.